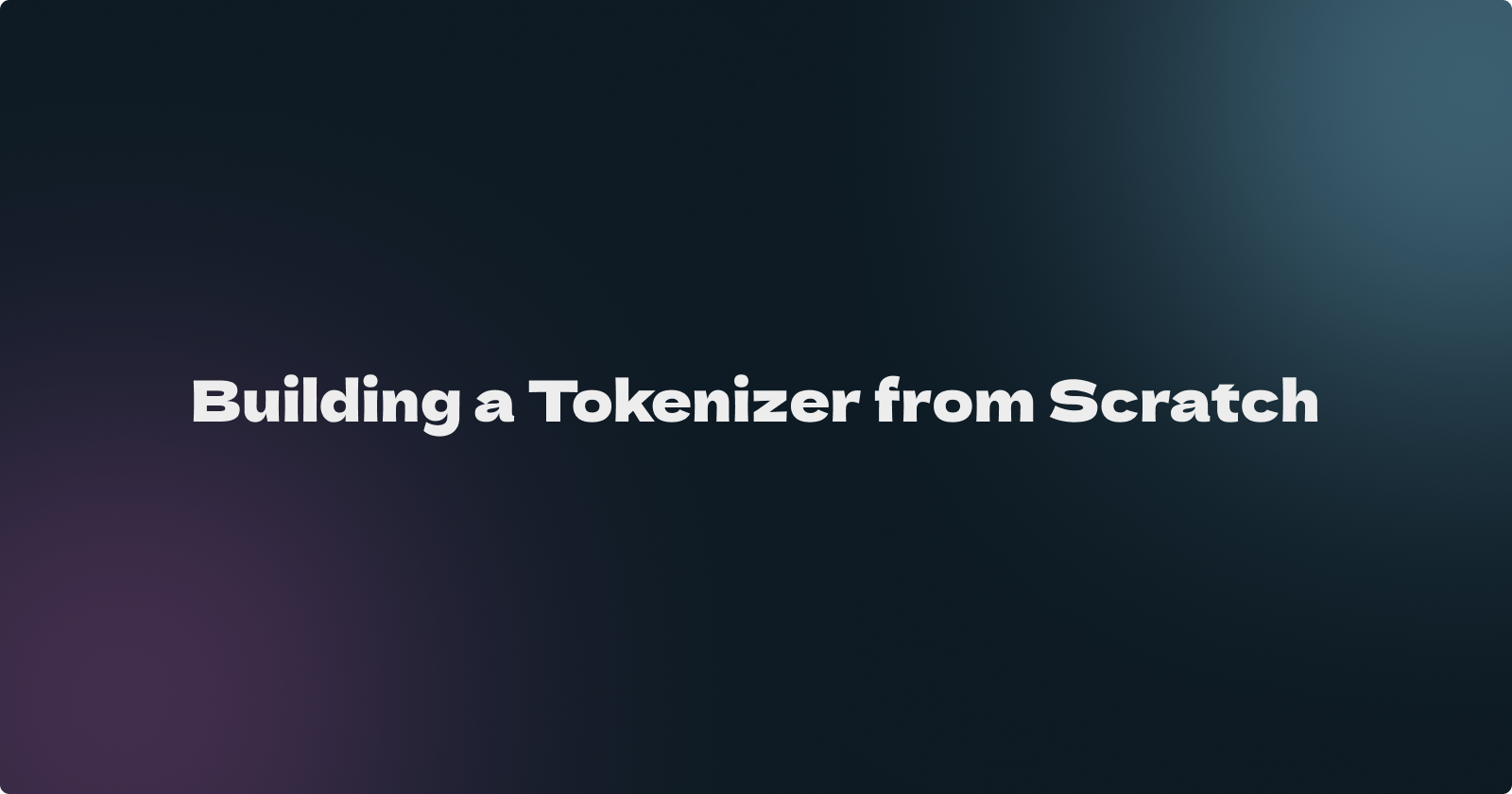
Building a Tokenizer from Scratch
Tokenization is a fundamental process in Natural Language Processing (NLP) that transforms raw text into numeric tokens that machine learning models can understand. In this blog post, I'll explain how to build a custom tokenizer, covering all the key concepts needed to understand modern language models.
What is a Tokenizer?
A tokenizer breaks down text into smaller units called tokens. These can be words, subwords, or characters depending on the strategy used. For language models like BERT or GPT, tokenization is the first step in processing any text input.
Key Concepts in Modern Tokenizers
1. Transformers
Transformers are a neural network architecture introduced in the paper "Attention Is All You Need" (2017). They revolutionized NLP by replacing recurrent neural networks with attention mechanisms. Tokenizers are the front door to transformer models, preparing text in a way transformers can process efficiently.
2. Encoder-Decoder Architecture
Encoder
The encoder processes the input sequence and creates a representation that captures the context of each token. For tokenization, encoders convert tokens to their numerical representations.
def encode(text, vocab):
tokens = tokenize(text)
return [vocab[token] for token in tokens]
Decoder
The decoder does the reverse job, converting numerical representations back to text.
def decode(ids, vocab_inverse):
return ' '.join([vocab_inverse[id] for id in ids])
3. Vector Representations
Tokens are converted into vectors (numerical arrays) to be processed by neural networks. These vectors capture the semantic properties of words.
4. Embeddings
Embeddings are dense vector representations of tokens where similar words have similar vectors. They allow models to understand semantic relationships between words.
# Example of simple embedding lookup
def get_embedding(token_id, embedding_matrix):
return embedding_matrix[token_id]
5. Positional Encoding
Since transformers process all tokens simultaneously (not sequentially), they need positional encoding to understand word order. Positional encodings are added to token embeddings to give the model information about token positions.
def positional_encoding(position, d_model):
# Create position-dependent encoding
angle_rates = 1 / np.power(10000, (2 * (np.arange(d_model) // 2)) / d_model)
angle_rads = position * angle_rates
# Apply sine to even indices, cosine to odd indices
pos_encoding = np.zeros(d_model)
pos_encoding[0::2] = np.sin(angle_rads[0::2])
pos_encoding[1::2] = np.cos(angle_rads[1::2])
return pos_encoding
6. Semantic Meaning
Tokenizers must preserve semantic meaning. This is challenging because different languages have different semantic structures and tokenization strategies must account for these variations.
7. Self-Attention
Self-attention allows models to weigh the importance of different tokens in a sequence when producing a representation for a specific token. This is crucial for understanding context.
def self_attention(query, key, value):
# Compute attention scores
scores = query @ key.transpose(-2, -1)
# Scale and apply softmax
scaled_scores = scores / math.sqrt(key.shape[-1])
weights = softmax(scaled_scores)
# Apply attention weights to values
return weights @ value
8. Softmax
Softmax normalizes a vector of numbers into a probability distribution. In tokenizers and transformers, it's used to convert attention scores into probabilities.
def softmax(x):
exp_x = np.exp(x - np.max(x, axis=-1, keepdims=True))
return exp_x / np.sum(exp_x, axis=-1, keepdims=True)
9. Multi-Head Attention
Multi-head attention allows models to focus on different parts of the input sequence simultaneously. Each "head" learns different aspects of the relationships between tokens.
10. Temperature
Temperature is a hyperparameter that controls the randomness of predictions. In tokenization, it can affect subword segmentation decisions.
11. Knowledge Cutoff
Knowledge cutoff refers to the limitation that models only have information up to their training cutoff date. Tokenizers need strategies to handle out-of-vocabulary words that appeared after the cutoff.
12. Tokenization Strategies
Different approaches to tokenization include:
- Word-based: Split text on whitespace/punctuation
- Character-based: Each character becomes a token
- Subword-based: Uses units smaller than words but larger than characters (used by BPE, WordPiece, SentencePiece)
A subword approach works best for many languages because:
- It handles morphological richness
- It manages out-of-vocabulary words
- It keeps vocabulary size manageable
13. Vocabulary Size
Vocabulary size refers to the number of unique tokens a tokenizer recognizes. Larger vocabularies capture more nuance but require more computation.
Implementing a Tokenizer
Here's a simplified approach to building a tokenizer:
- Collect a representative text corpus
- Learn subword units using algorithms like Byte-Pair Encoding (BPE)
- Create a vocabulary based on these units
- Implement encoding/decoding functions
- Add special tokens for sentence boundaries, padding, etc.
class CustomTokenizer:
def __init__(self, vocab_size=30000):
self.vocab_size = vocab_size
self.bpe_model = None
self.vocab = {}
self.inverse_vocab = {}
def train(self, corpus):
# Train BPE model on corpus
# Build vocabulary
pass
def encode(self, text):
# Convert text to token IDs
pass
def decode(self, ids):
# Convert token IDs back to text
pass
Conclusion
Building a tokenizer from scratch requires understanding several fundamental concepts in NLP and transformer architecture. By mastering these concepts, you can create an effective tokenizer that preserves semantic meaning, enabling better performance for downstream NLP tasks.
In my implementation, I focused on a subword approach using Byte-Pair Encoding which handles morphological differences well while maintaining a manageable vocabulary size. Feel free to experiment with different strategies and parameters to optimize for your specific use case!